#9 async & await - 직관적인 비 동기 처리 코드 작성하기
< async >
: Promise를 더 쉽게 이용할 수 있도록 도와줌
function hello() {
return "hello";
}
async function helloAsync() {
return "hello async";
}
console.log(hello()); //hello
console.log(helloAsync());
helloAsync().then((res) => {
console.log(res);
}) //hello async
< await 사용하지 않고 시간 기다리기 >
function delay(ms) {
return new Promise((resolve) => {
setTimeout(() => {
resolve();
}, ms);
});
}
async function helloAsync() {
return delay(3000).then(() => {
return "hello async";
})
}
helloAsync().then((res) => {
console.log(res);
}) //hello async
< await 사용하여 시간 기다리기 >
- await
: 비동기 함수가 동기적인 것처럼 작동
: async 내에서만 사용 가능
function delay(ms) {
return new Promise((resolve) => {
setTimeout(() => {
resolve();
}, ms);
});
}
async function helloAsync() {
await delay(3000);
return "hello async";
}
helloAsync().then((res) => {
console.log(res);
}) //hello async
async function main() {
const res = await helloAsync();
console.log(res);
}
main(); //hello async
#10 API 호출하기 - API & Fetch
< API: Application Programming Interface >
: 응용 프로그램 프로그래밍 인터페이스
: 응용 프로그램에서 사용할 수 있도록 운영체제나 프로그래밍 언어가 제공하는 기능을 제어하도록 만든 인터페이스
: 주로 파일 제어, 창 제어, 화상 처리, 문자 제어 등을 위한 인터페이스 제공
: 다른 프로그램에게 데이터를 받기 위한 호출
손님 | 1. 주문 → | 웨이터 | 2. 찾기 → | 냉장고 |
← 4. 음식 서빙 | ← 3. 가져오기 |
Client | 1. Request → | Server | 2. Query → | DataBase |
← 4. Response | ← 3. Query Result |
< API 호출 >
- Json Placeholder에 접속 후 링크 복사
let response = fetch('https://jsonplaceholder.typicode.com/posts').then((res) => {
console.log(res);
})
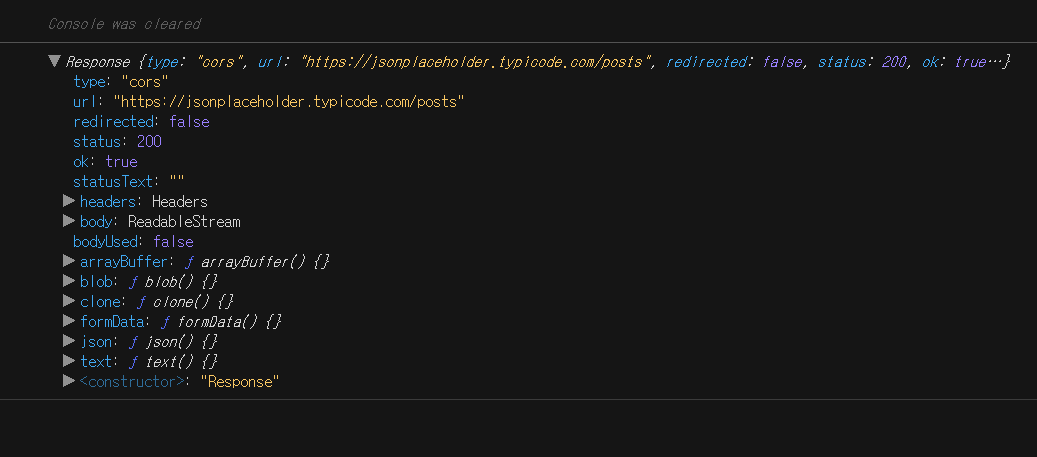
async function getData() {
let rawResponse = await fetch('https://jsonplaceholder.typicode.com/posts');
let jsonResponse = await rawResponse.json();
console.log(jsonResponse);
};
getData();
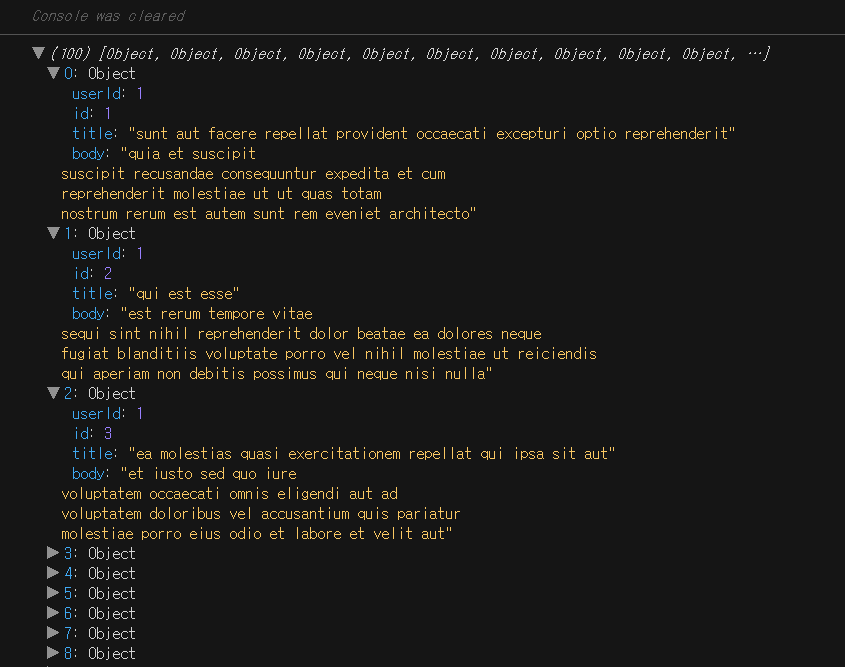
이정환님의 인프런 강의 "한입 크기로 잘라 먹는 리액트(React.js) : 기초부터 실전까지"를 참고하여 작성하였습니다.
'Web > JavaScript' 카테고리의 다른 글
JavaScript 응용 #4 Promise - 콜백 지옥에서 탈출하기 (0) | 2023.09.16 |
---|---|
JavaScript 응용 #3 동기 & 비동기 (0) | 2023.09.16 |
JavaScript 응용 #2 조건문, 비 구조화 할당, Spread 연산자 (0) | 2023.09.14 |
JavaScript 응용 #1 Truthy & Falsy, 삼항 연산자, 단락회로 평가 (0) | 2023.09.14 |
JavaScript 기본 #4 배열 내장 함수 (0) | 2023.09.10 |